SQL Joins are useful concepts for working with relational data. Joins allow you to combine data from two or more tables based on related columns. Using joins you can create effective database queries. In this blog, we’ll explore inner joins, left joins, right joins, full joins, cross joins, and self joins. By the end, you’ll have a solid understanding of using joins to manage and query relational data.
What Are SQL Joins?
SQL joins are used to retrieve data from two or more tables based on a related column. Since relational databases are designed to store data in separate tables. Joins are crucial for combining this data for analysis.
Why Learn SQL Joins?
- Joins simplify data aggregation across related tables.
- They are essential for real-world database tasks, from e-commerce applications (linking customers with orders) to financial analysis (linking transactions with accounts).
- Understanding joins can greatly enhance query efficiency and readability.
Let’s dive into each type of SQL join with examples and diagrams.
Example Tables: Customers and Orders
We’ll use two tables, Customers and Orders, to demonstrate each join type.
Customers Table
CREATE TABLE Customers (
CustomerID INT PRIMARY KEY,
Name VARCHAR(50),
City VARCHAR(50)
);
INSERT INTO Customers VALUES
(1, 'Oliver', 'New York'),
(2, 'Jack', 'Los Angeles'),
(3, 'Harry', 'Chicago'),
(4, 'Jacob', 'Houston');
Orders Table
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
CustomerID INT,
Amount DECIMAL(10, 2)
);
INSERT INTO Orders VALUES
(1, 1, 100.00),
(2, 2, 200.00),
(3, 2, 350.00),
(4, 5, 500.00);
Inner Join
An inner join retrieves rows with matching values in both tables.
Use Case
Retrieve all customers who have placed orders.
Query
SELECT Customers.Name, Orders.Amount
FROM Customers
INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Output
Name | Amount |
Oliver | 100.00 |
Jack | 200.00 |
Jack | 300.00 |
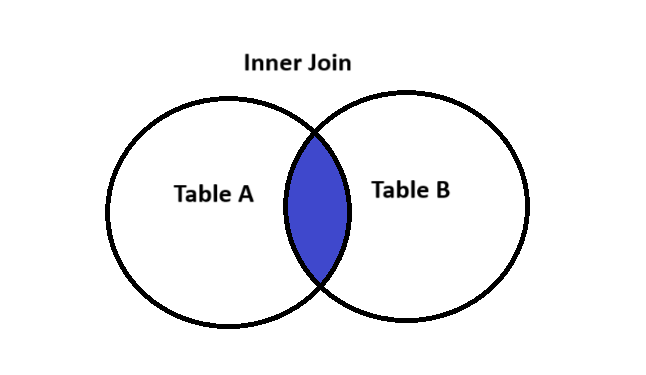
Left Join (Left Outer Join)
A left join retrieves all rows from the left table and matching rows from the right table. If no match is found, NULLs are returned.
Use Case
Retrieve all customers, including those who haven’t placed orders.
Query
SELECT Customers.Name, Orders.Amount
FROM Customers
LEFT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Output
Name | Amount |
Oliver | 100.00 |
Jack | 200.00 |
Jack | 350.00 |
Harry | NULL |
Jacob | NULL |
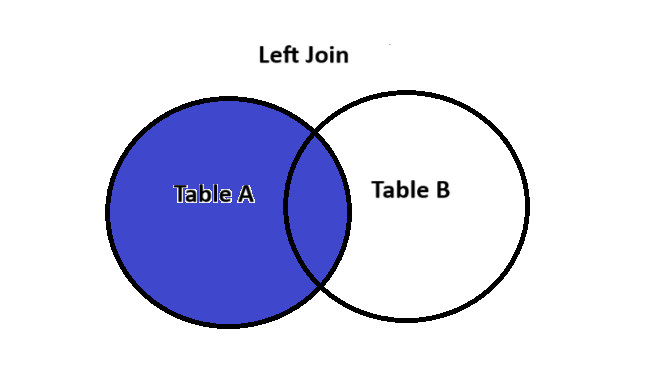
Right Join (Right Outer Join)
A right join retrieves all rows from the right table and matching rows from the left table. If no match is found, NULLs are returned.
Use Case
Retrieve all orders, including those not linked to customers.
Query
SELECT Customers.Name, Orders.Amount
FROM Customers
RIGHT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Output
Name | Amount |
Oliver | 100.00 |
Jack | 200.00 |
Jack | 350.00 |
NULL | 500.00 |
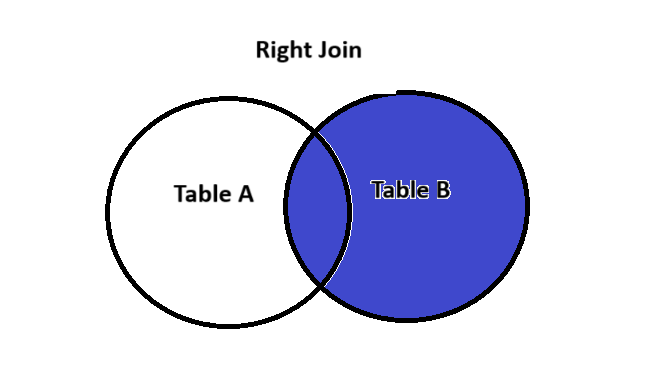
Full Join (Full Outer Join)
A full join retrieves all rows from both tables. Non-matching rows are filled with NULLs.
Use Case
Retrieve all customers and all orders, whether or not they match.
Query
SELECT Customers.Name, Orders.Amount
FROM Customers
FULL JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Output
Name | Amount |
Oliver | 100.00 |
Jack | 200.00 |
Jack | 350.00 |
Harry | NULL |
Jacob | NULL |
NULL | 500.00 |
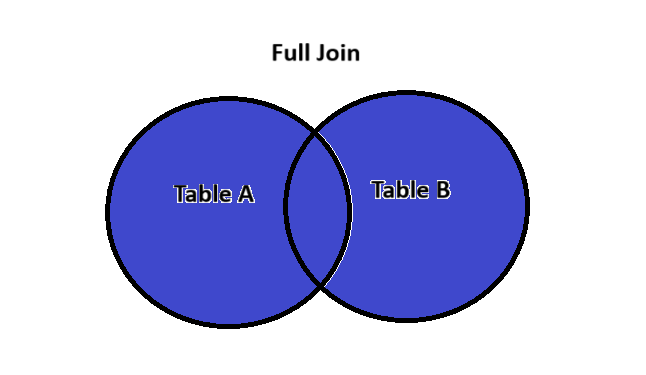
Cross Join
A cross join produces a Cartesian product of both tables.
Use Case
Generate all possible combinations of customers and orders (e.g., for testing).
Query
SELECT Customers.Name, Orders.Amount
FROM Customers
CROSS JOIN Orders;
Output
Name | Amount |
Oliver | 100.00 |
Jack | 100.00 |
Harry | 100.00 |
Jacob | 100.00 |
Oliver | 200.00 |
Jack | 200.00 |
Harry | 200.00 |
Jacob | 200.00 |
Oliver | 350.00 |
Jack | 350.00 |
Harry | 350.00 |
Jacob | 350.00 |
Oliver | 500.00 |
Jack | 500.00 |
Harry | 500.00 |
Jacob | 500.00 |
Self Join
A self join joins a table to itself.
Use Case
Find customers in the same city.
Query
SELECT A.Name AS Customer1, B.Name AS Customer2
FROM Customers A
JOIN Customers B ON A.City = B.City AND A.CustomerID != B.CustomerID;
Best Practices and Optimization Tips
- Indexing: Add indexes to columns used in joins for faster query execution.
- Avoid Unnecessary Joins: Only include tables and columns needed for the query.
- Filter Early: Use WHERE conditions to reduce the dataset size before joining.
- Analyze Execution Plans: Use tools like SQL Server Management Studio (SSMS) to identify bottlenecks.
Conclusion
SQL joins are powerful tools for working with relational data. Mastering inner, left, right, full, cross, and self joins can unlock your databases’ full potential. Remember to use best practices to optimize your queries for performance and efficiency.
Quick Reference Guide:
- Inner Join: Matches rows in both tables.
- Left Join: Includes all rows from the left table.
- Right Join: Includes all rows from the right table.
- Full Join: Includes all rows from both tables.
- Cross Join: Produces all possible combinations.
- Self Join: Joins a table to itself.
With these techniques, you’re ready to tackle any SQL join challenge!