Middleware is an important part of .NET Core applications. It provides a way to handle requests and responses within the web application pipeline. If you want to understand middleware better, this blog is for you. We will cover what middleware is, why it is important, and how you create your custom middleware with examples.
Introduction
In web development, handling HTTP requests and responses efficiently is important. Middleware in .NET Core plays a key role in this process. Middleware components are small, modular pieces of software that process requests and responses. Think of middleware as a pipeline where each component can inspect, modify, or terminate requests and responses as they flow through.
Middleware can perform tasks such as:
- Logging requests
- Handling authentication
- Serving static files
- Redirecting URLs
In this blog, we’ll dive into the concept of middleware, explore built-in middleware in .NET Core, and create custom middleware to demonstrate how robust and flexible this system can be.
What is Middleware?
Middleware are software components that are assembled into an application pipeline to handle requests and responses. Each component in the pipeline decides whether to pass the request to the next component or handle it directly.
When an HTTP request comes into a .NET Core application, it travels through a sequence of middleware components before reaching the endpoint that generates the response. The response then travels back through the same sequence of middleware components.
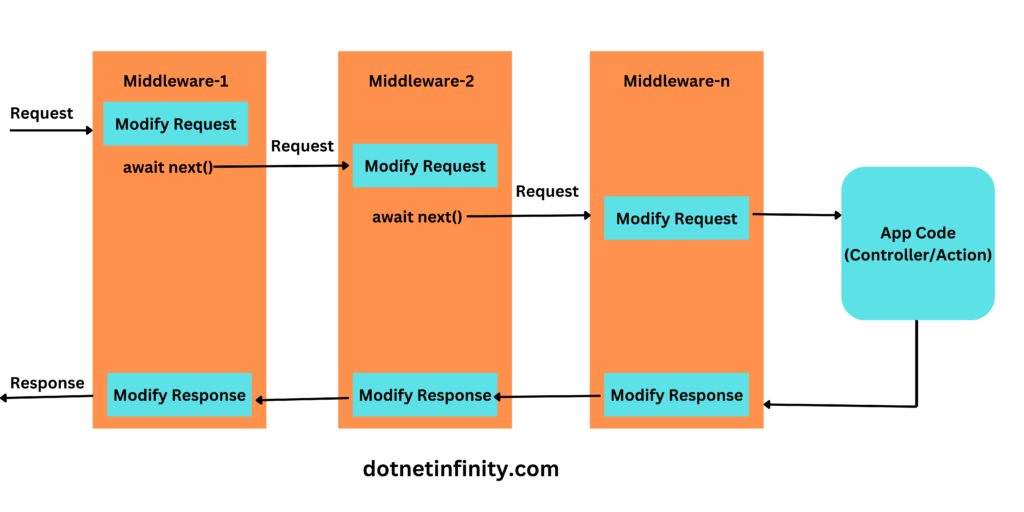
Key Characteristics of Middleware
1. Order Matters: The order in which middleware is added affects how requests and responses are processed.
2. Short-Circuiting: Middleware can decide to terminate the request pipeline early, preventing further processing.
For ex. a) It checks if a user is authorized to access a resource. If it is not authorized then it will return an appropriate response and it will prevent further processing.
b) Suppose we have created a custom middleware for API that checks the presence of the valid header. If the valid header is missing, the middleware will short-circuit the pipeline and return a 400 Bad Request response.
Therefore it is the most important characteristic of middleware. Short-circuiting is the most asked question in Interview 🙂
3. Dependency Injection: Middleware can use dependency injection to get the required services.
Built-in Middleware in .NET Core
.NET Core has various built-in middleware that you can use out of the box. Here are some common ones:
- Static Files Middleware: Serves static files such as HTML, CSS, JavaScript, and images.
- Routing Middleware: Matches request URLs to configured routes.
- Authentication Middleware: Handles user authentication.
- Authorization Middleware: Checks if the user is authorized to access a resource.
Example: Using Static Files Middleware
To use the Static Files Middleware, you simply add it to the pipeline in the Startup.cs file:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseStaticFiles(); // Enables serving static files from wwwroot
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
Creating Custom Middleware
Creating custom middleware allows you to add unique functionality to your application pipeline. Let’s understand this with an example. We have discussed the above most important characteristic of middleware is Short-Circuiting, so let’s understand this by creating custom middleware.
Example: Creating Short-Circuiting Middleware
Let’s create a custom middleware that checks for the presence of a specific header in the request. If the header is absent, the middleware will return a 400 Bad Request response and short-circuit the pipeline.
Step-by-Step Guide to Creating Custom Middleware
1) Create a Middleware Class
First, create a new class for your middleware. This class needs a constructor to accept a RequestDelegate and an InvokeAsync method to handle the request.
This class will check for the presence of the X-Valid-Header header and short-circuit the pipeline if the header is missing.
public class HeaderValidationMiddleware
{
private readonly RequestDelegate _next;
public HeaderValidationMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context)
{
if (!context.Request.Headers.ContainsKey("X-Valid-Header"))
{
context.Response.StatusCode = 400; // Bad Request
await context.Response.WriteAsync("Missing X-Valid-Header");
return; // Short-circuit the pipeline
}
await _next(context); // Call the next middleware in the pipeline
}
}
2) Register Middleware in Startup.cs
Add the custom middleware to the pipeline in the Configure method of Startup.cs:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMiddleware<HeaderValidationMiddleware>(); // Add header validation middleware
app.UseStaticFiles();
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
3) Testing the Middleware
Run your application and make some requests. You can use tools like Postman or your browser’s developer tools to test the presence or absence of the X-Valid-Header header. If you don’t have Postman, click here to download it.
a) Request with Header
If you include the X-Valid-Header header in your request, the request will proceed through the rest of the middleware pipeline and eventually reach the endpoint.
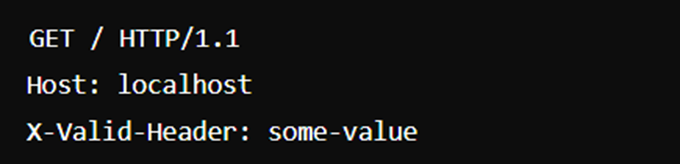
Response:
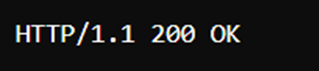
b) Request without Header
If you do not include the X-Valid-Header header in your request, the middleware will short-circuit the pipeline and return a 400 Bad Request response.
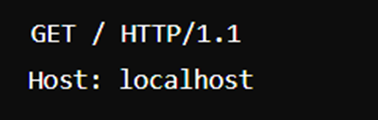
Response:
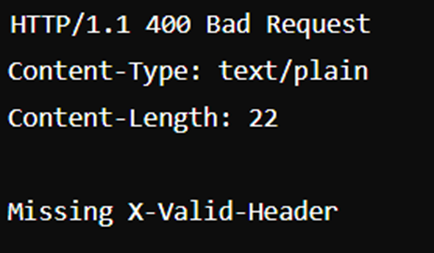
Conclusion
Middleware is a powerful feature in .NET Core that allows you to handle requests and responses flexibly and modularly. If you understand middleware, it will greatly enhance your application’s functionality and maintainability.
In this blog, we’ve covered the basics of middleware, explored some built-in middleware components, and demonstrated how to create custom middleware with practical examples.
Whether logging requests, adding headers or handling authentication, middleware is an essential tool for building robust and efficient web applications in .NET Core. Start experimenting with middleware today, and you’ll soon see the benefits it brings to your development workflow.